JavaScript is a fundamental programming language that is crucial to web development. Whether you’re new to coding or a seasoned developer, having a comprehensive JavaScript cheat sheet pdf can be incredibly helpful. This guide offers a detailed reference to JavaScript’s key concepts, best practices, and essential tips.
JS Cheat Sheet pdf
JavaScript is a versatile, high-level programming language primarily used in web development. Moreover, it allows developers to create interactive and dynamic web pages, thus making it an integral part of modern web development. JavaScript plays a pivotal role in enhancing web pages by facilitating user interaction, form validation, animations, and real-time updates. It operates in web browsers and developers can also utilized on the server side through technologies like Node.js.
Understanding JavaScript syntax is essential. JavaScript employs a C-style syntax, featuring elements such as variables, functions, and control structures. Semicolons terminate statements, while curly braces define code blocks.
JS cheat sheet pdf file is attached here and the key features are:
Data Types and Variables
Variables and Their Purpose: Variables are used to store and manage data in JavaScript, serving as containers for values. JavaScript offers three ways to declare variables such as var, let, and const, each with its specific use cases.
Common Data Types in JavaScript: JavaScript supports various data types such as:
- String: This represents textual data enclosed within quotation marks.
- Number: Handles numeric values.
- Boolean: Stores true or false values.
- Array: Organizes collections of data in an ordered manner.
- Object: Represents data structures containing key-value pairs.
- Undefined: Signifies the absence of an assigned value.
- Null: Denotes the intentional absence of any object or value.

JavaScript Data Types
Converting Data Types: JavaScript provides methods and functions to convert data from one type to another. For instance, you can convert a string to a number using functions like parseInt() and parseFloat(). Alternatively, you can change the type of a variable explicitly using String().
You can download JavaScript Cheat Sheet PDF.![]() |
Operators
Arithmetic Operators: Arithmetic operators perform mathematical operations like addition (+), subtraction (-), multiplication (*), division (/), and modulus (%).
Comparison Operators: Comparison operators are used to compare values and determine their relationship. Common comparison operators include equality (==), strict equality (===), inequality (!=), strict inequality (!==), and greater than (>), among others.
Logical Operators: Logical operators are used to combine or modify logical values. The primary logical operators in JavaScript are logical AND (&&), logical OR (||), and logical NOT (!).
Assignment Operators: Assignment operators are employed to assign values to variables. Common assignment operators include the assignment operator (=), addition assignment (+=), and subtraction assignment (-=).
Control Flow
Conditional Statements (if, else if, else): Conditional statements in JavaScript allow you to execute specific code blocks based on specified conditions. People mostly use if, else if, and else statements for branching logic.
The Switch Statement: The switch statement is another conditional statement that simplifies complex branching logic. It evaluates an expression and executes a block of code based on the value of that expression.
Loops (for, while, do…while): Loops are used to execute a block of code repeatedly. JavaScript provides several loop structures, including for, while, and do…while, each suitable for different scenarios.
Using Break and Continue: To exit a loop prematurely, use the break statement. On the other hand, the continue statement skips the current iteration and moves to the next one within the loop.
Functions
Functions Explained: Functions in JavaScript are reusable blocks of code that perform specific tasks or calculations. They allow you to encapsulate logic and execute it whenever needed. Function parameters are placeholders for values that the function expects as input. They can take parameters as input and return values as output.
Function Parameters and Return Values: Function parameters are placeholders for values that the function expects as input. Functions can also return values, which represent the output or result of the function’s operation.
Function Expressions and Arrow Functions: JavaScript supports various ways to define functions, including function declarations, function expressions, and arrow functions. Function expressions can be assigned to variables and passed as arguments to other functions.
Arrays
In JavaScript, developers use arrays as data structures to store and manage collections of values in an ordered manner. Consequently, they make it easy to organize data and manipulate elements within the collection.
Accessing and Modifying Array Elements: Arrays are zero-indexed, meaning the first element is accessed with an index of 0. You can access and modify array elements using square brackets and their corresponding indices.
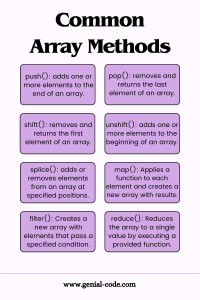
Common Array Methods
Common Array Methods: JavaScript provides a range of built-in methods for working with arrays, including common methods such as:
- push(): This method adds one or more elements to the end of an array.
- pop(): This method removes and returns the last element of an array.
- shift(): This method removes and returns the first element of an array.
- unshift(): This method adds one or more elements to the beginning of an array.
- splice(): This method adds or removes elements from an array at specified positions.
Objects
Introduction to Objects: Objects in JavaScript are complex data structures that store and organize key-value pairs. They play a central role in many applications and are a fundamental concept in the language.
Properties of Objects: Objects consist of properties, which are key-value pairs. You can access properties using dot notation (e.g., objectName.propertyName) or bracket notation (e.g., objectName[‘propertyName’]).
Methods in Objects: Objects can also possess methods, associating functions with the object. Methods that allow objects to perform actions and calculations.
Object Destructuring: Object destructuring, a feature in JavaScript, enables you to extract specific properties from an object and assign them to variables. Consequently, this approach offers a convenient way to work with objects and access their values.
Scope and Closures
The term “Scope” in JavaScript refers to the specific context or environment in which variables are declared and accessed within a program. Consequently, it is crucial to understand the scope for managing variable lifecycles and preventing conflicts.
Closures are a powerful concept in JavaScript. They occur when a function retains access to variables from its containing (enclosing) function’s scope, even after the containing function has finished executing. People often use closures to create private variables and maintain state.
Error Handling
Error handling is an essential part of programming. Therefore in JavaScript, you can use try…catch statements to gracefully handle errors and prevent them from crashing your application. The try…catch statement allows you to enclose code that might throw an exception (an error) within a try block.
Asynchronous JavaScript
JavaScript is inherently asynchronous, that means it can execute multiple operations simultaneously without blocking the main thread. So, this is crucial for tasks like handling user input, making network requests, and performing animations.
Leave a Comment