Flutter is a powerful framework that enables developers to create native-like user interfaces for multiple platforms using a single codebase. It has gained popularity for its fast development capabilities and expressive UI. Flutter is known for its hot-reload feature, which allows developers to see real-time changes in their app as they code, making the development process highly efficient. This Beginning Flutter handwritten notes Guide may help you to learn the basics of Flutter.
Flutter Handwritten Notes key advantages:
- Cross-Platform Development: Flutter enables cross-platform app development for iOS, Android, web, and desktop, reducing development time and maintaining consistent user experiences.
- Expressive UI: Flutter provides a wide range of customizable widgets, allowing developers to create stunning and highly interactive user interfaces. The widgets are designed to look and feel native on each platform.
- Hot Reload: Flutter’s hot reload allows developers to view real-time code updates in the app that improve productivity and speeding up debugging.
- High Performance: Flutter apps are compiled into native ARM code, resulting in faster startup times, responsive user interfaces, and smooth animations.
- Strong Community: Flutter’s thriving developer community contributes to its growth with numerous packages and plugins available to extend app functionality.
- Open-Source: Flutter is open-source and free to use, making it accessible to developers worldwide. Its open nature encourages collaboration and innovation within the community.
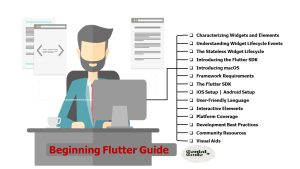
Beginning Flutter Guide
Flutter Architecture
To understand Flutter programming better, let’s delve into its architecture:
- Flutter Framework: At the core of Flutter is the framework, which provides a wide range of widgets, libraries, and tools for building user interfaces and handling application logic. The framework is written in Dart, a programming language also developed by Google.
- Dart Language: Flutter apps are primarily developed using the Dart programming language, which is fast and easy to learn for developers familiar with Java or JavaScript.
- Widgets: Flutter apps are built using widgets, that range from simple buttons to complex layouts. There are two types of widgets: StatelessWidget, which is immutable, and StatefulWidget, which is mutable. You can compose widgets to create complex UIs.
- Rendering Engine: Flutter has its rendering engine responsible for rendering the widgets on the screen, that provides high-performance graphics and smooth animations, making Flutter apps feel native.
- Platform Channels: Flutter communicates with the native platform via platform channels. These channels allow the Flutter code to interact with the underlying platform-specific code to perform tasks such as accessing device hardware or integrating with platform-specific features.
Start with Flutter Programming
Let’s dive into Flutter programming with some code examples. First, you need to set up Flutter on your development machine:
1. Installing Flutter:
Download the Flutter SDK for your operating system from the Flutter website (https://flutter.dev), and follow the installation instructions to add Flutter to your system’s PATH.
2. Creating a Flutter Project:
To create a new Flutter project, open your terminal and run the following commands:
This will create a new Flutter project with a default directory structure.
3. Writing Your First Flutter Code:
Let’s create a simple “Hello, Flutter!” app. Open the lib/main.dart file in your project directory and replace the existing code with the following:
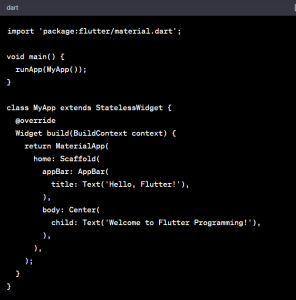
Hello Flutter App
This code defines a basic Flutter app with a Material Design structure. It displays an app bar with a title and a centered text on the screen.
4. Running Your App:
To see your app in action, open your terminal, navigate to your project’s root directory, and run:

Run Flutter App
This command will launch your app on an emulator or physical device, and you’ll see the “Hello, Flutter!” app in action.
5. Hot Reload:
Let’s make use of one of Flutter’s most useful features – hot reload. You can take advantage of this feature while your app is running on either an emulator or a physical device. Simply modify the text inside the Text widget – for example, you could change ‘Welcome to Flutter Programming!’ to ‘Flutter is amazing!’ – and save the file. Immediately, you’ll see the updated text displayed in your app without needing to restart it. This feature makes the development process more efficient and allows for rapid iteration.
Beginning Flutter Guide Widgets
Flutter provides an extensive library of widgets, each serving a specific purpose in crafting user interfaces. Commonly used widgets are:
- Text Widget: Display text with various styles, fonts, and formatting options.
- Container Widget: A versatile widget for creating boxes with customizable properties like background color, borders, and padding.
- Row and Column Widgets: Layout widgets facilitate the arrangement of child widgets horizontally or vertically, aiding in creating responsive UIs.
- Image Widget: Display images from various sources, including assets, network URLs, and the device’s gallery.
- ListView Widget: Construct scrollable lists of items, which can be oriented vertically or horizontally, allowing for the creation of various UI patterns like lists and carousels.
- AppBar Widget: A customizable app bar typically positioned at the top of the screen, which often contains app-specific actions, navigation controls, and branding.
- Button Widgets: Flutter offers a variety of buttons, such as RaisedButton, FlatButton, and IconButton, allowing for the inclusion of interactive elements in your UI.
- TextField Widget: Input fields for capturing user text input, with customizable properties for validation, input masks, and more.
- AlertDialog Widget: Display dialog boxes with messages, options, and actions to interact with the user.
- GestureDetector Widget: Detect and respond to user gestures, such as taps, swipes, and drags, enabling the implementation of interactive user interfaces.
Beginning Flutter Guide offers a powerful solution for developing cross-platform apps with remarkable efficiency. Its expressive UI capabilities, hot reload feature, and active community support make it a compelling choice for developers. As you explore Flutter further, you’ll discover its flexibility and versatility in crafting beautiful and performant apps across mobile, web, and desktop platforms. The code examples provided here are just the tip of the iceberg, and there’s much more to explore in the world of Flutter programming.
Leave a Comment